Clobbering Together a Secure Online Resume Viewer
Unix is simple. It just takes a genius to understand its simplicity.
, Dennis Ritchie
18 Oct 2020
Introduction
In recent years, there is a growing awareness of online privacy and cybersecurity. Governments have passed laws to protect user data and guard the privacy of their citizens. In Singapore, there is the Personal Data Protection Act (PDPA) which seeks to protect the personal data of an individual. An individual can also take some common sense precautions such as not giving out or posting sensitive personal data online, to ensure his or her own privacy.
Many social media platforms, such as professional networking and career sites, job hunting sites etc... often collect a fair amount of personal data. Technical people may also have their own websites and post their resumes online. For those who are concerned about data protection and privacy, one approach is to have your own secure online resume viewer, granting access only to those you trust. This article shows how to clobber together a simple secure resume viewer.
Design and Approach
We will use php to create the resume viewer. The format of the resume file itself is assumed to be html 5, since it is supposed to be an online resume. CSS stylesheet can be used to provide a nice layout and presentation of the resume file.
Security is important, so the Php resume viewer script needs to have a login. We need to have a way to store the user accounts and passwords. We can also have multiple resume files catered to specific users. For example, we can have a generic resume that we give out to tech recruiters in general. We can also have more specialized resumes, targetting positions that we are interested in.
Each user that logs in will get to see a particular resume file. We may also want to track statistics, like when a user has logged in and when a resume is viewed. This can be done through many different means, but it may be useful to have a backend database for this.
Not everybody will want to run a database just for a simple php script. Well, the script needs to have some toggling flags that turn on or off the database feature. Another important security feature is logging, we need to log failed logins and potentially send out email alerts.
Sending out email alerts require a properly setup smtp server. Again not everyone will have one ready. So toggling flag can be used to enable or disable email alerts. To prevent brute force attempts at guessing credentials, we can set up a captcha system or maybe use a rate limiting system, or have both of them.
For a website that doesn't have a ready captcha script/app available, we can have toggling flag that enables or disable captcha. We also want a feature that will automatically log out a recruiter or hr personnel if no activity is detected for a specific time. A recruiter may have logged in and left the computer to run some other errands. For better security and privacy, we don't want our resume to be unnecessarily exposed. This is just like a enabling screensaver for the computer screen. Javascript can be used for such protection.
By now we have accumulated a list of requirements for our resume viewer script. A simple use case narration that describes what an application should do, can quickly ellicit the functionalities needed. The following summarizes the key requirements.
- User and password login is required
- Each user can view a specific resume file
- Proper statistics, logging and email alerts can be enabled
- Captcha can be enabled
- Timeout to auto log out a user after a specific time
The next section will explain the implementation in detail.
Implementation
Before we see the code for the main resume viewer, we need to create a utility first. As our resume viewer requires a user to log in, we need to store the password of each user. We don't want it to be stored in the clear. A good choice is to hash it using an algorithm like bcrypt.
To create this bcrypt hash, a utility is required. The following bcrypthash.php is a simple web based form that can create the password hash. We enter a desired password and submit the form, it will return the corresponding bcrypt hash, together with its random salt. This entire hash string can be used to verify the user password later.
The utility uses php password_hash() function to create the bcrypt hash. We let the function automatically creates the random salt. The php password_verify() function can be used to verify against the plain text password against the hash string that is created.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | <?php
/* Allow only GET and POST */
if(strcasecmp("post", $_SERVER['REQUEST_METHOD']) != 0 && strcasecmp("get", $_SERVER['REQUEST_METHOD']) != 0)
{
error_log("Invalid HTTP method : " . $_SERVER['REQUEST_METHOD'] . " : " . $remoteip ,0);
die();
}
header('Content-Type: text/html; charset=UTF-8');
header('Cache-control: no-store');
header('X-Content-Type-Options: nosniff');
header('X-Frame-Options: DENY');
header('X-XSS-Protection: 1; mode=block');
header('Content-Security-Policy: default-src \'self\'; frame-ancestors \'self\'; base-uri \'self\'; form-action \'self\'; report-uri /csprp/; ');
header('Referrer-Policy: same-origin');
/* Handle POST */
if(strcasecmp("post", $_SERVER['REQUEST_METHOD']) == 0 )
{
if(!isset($_POST['plainpassword']))
{
echo "Plain password not set !";
error_log("Plain password not set !",0);
die();
}
$plain_password = $_POST['plainpassword'];
$hash_pass = password_hash($plain_password, PASSWORD_BCRYPT);
if(!$hash_pass)
{
echo "Error generating bcrypt hash!";
error_log("Error generating bcrypt hash!",0);
die();
}
echo $hash_pass;
exit(0);
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Php Password Hash Utility</title>
</head>
<body>
<h2>Generates Php BCRYPT hashes</h2>
<p>Note there is no check on password length and complexity. Choose and use a complex and sufficiently long password yourself.
Use only ASCII characters, numeric digits, various punctuation marks, numeric operators. The php password_hash method is not binary safe.
</p>
<div>
<form action="bcrypthash.php" accept-charset="utf-8" method="post" enctype="application/x-www-form-urlencoded">
<label>Enter Password</label><br>
<input type="text" name="plainpassword" placeholder="Plain text password" maxlength="50" size="30" required>
<br><br>
<input type="submit" value="Generate Bcrypt Hash" >
</form>
</div>
</body>
</html>
|
Notice that our php script sets a number of web security headers, including no-store for Cache-control and a Content Security Polcy that prevents inline javascript. Although the script itself doesn't have javascript injection vulnerability, it is still good practice to set up a set of web security headers.
The following screenshot shows how the bcrypthash.php looks like in actual use.
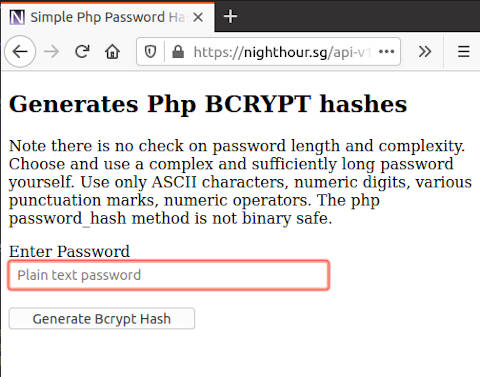
After submitting a password, it will return the bcrypt hash string.
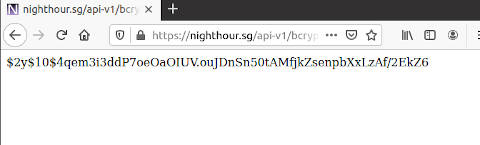
This bcrypt hash string will be used in our main resume viewer when defining the user login credential.
The following shows index.php, the script file of our online resume viewer.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 | <?php
class User
{
public $userid;
public $password_hash;
public $file;
function __construct($userid, $password_hash, $file)
{
$this->userid = $userid;
$this->password_hash = $password_hash;
$this->file = $file;
}
}
$valid_users = array (
"hr01" => new User("hr01", '$2y$10$OoeJGygHV0eP8VLuiiGzFuI/UzpkV3PAKPB2RexhUcWOzjx7g2lGG', '/opt/resume/resume.html'),
"hr02" => new User("hr02", '$2y$10$tXRE07sSvAdOlxZyyZLgceozQuzC0QCiPcXqyZIrN9ec.G3NtvKxi', '/opt/resume/resume-10-20.html'),
"hr03" => new User("hr03", '$2y$10$lPnIVt5kBRoJHgxLtb0h5u8vK8Nw3FKEY5ox38xlbNinkZx1PSZR2', '/opt/resume/resume-10-12.html'),
"myuser01" => new User("myuser01", '$2y$10$KVOfnXtbU3A4GA7cqKgL/e5s9Nxn/MtFxBCA6lJUrEky8ptVKAULq', '/opt/resume/resume-10-15.html')
);
$db_enable = true;
$em_enable = true;
$captcha_enable = true;
$email_account = "my-special-alert001@nighthour.sg";
$rurl = "https://nighthour.sg/resume/";
$relative_url = "/resume/";
$captcha_timeout = 900;
$max_session_time = 1800; // maximum view time 30 minutes
$session_timeout = 300; //time out after 5 mins of no activity
function getDatabasePDO()
{
$host = '127.0.0.1';
$db = 'resumedb1';
$user = 'resumeuser01';
$pass = 'XjDxIzdk#V8>Q%+9a!1BVqceFusFP-qN@dQgNE3uVy4JbULm';
$charset = 'utf8';
$dsn = "mysql:host=$host;dbname=$db;charset=$charset";
$opt = [
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION,
PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC,
PDO::ATTR_EMULATE_PREPARES => false,
PDO::MYSQL_ATTR_SSL_KEY =>'/opt/phpcerts/client-key.pem',
PDO::MYSQL_ATTR_SSL_CERT=>'/opt/phpcerts/client-cert.pem',
PDO::MYSQL_ATTR_SSL_CA =>'/opt/phpcerts/ca-cert.pem',
PDO::MYSQL_ATTR_SSL_VERIFY_SERVER_CERT => false
];
$pdo = new PDO($dsn, $user, $pass, $opt);
return $pdo;
}
/* Update views into the database */
function updateDBView($ip, $username, $filename)
{
global $db_enable;
if(!$db_enable)
{
return;
}
$pdo = getDatabasePDO();
$stmt = $pdo->prepare('INSERT INTO resumeview (ip,username,file) values(?,?,?)');
$ret = $stmt->execute([$ip, $username, $filename]);
$stmt = null;
$pdo = null;
return $ret;
}
/* Update fail login attempt into the database */
function updateDBFailLogin($ip, $username, $password)
{
global $db_enable;
if(!$db_enable)
{
return;
}
$pdo = getDatabasePDO();
$stmt = $pdo->prepare('INSERT INTO failedlogin (ip,username,password) values(?,?,?)');
$ret = $stmt->execute([$ip, $username,$password]);
$stmt = null;
$pdo = null;
return $ret;
}
/* Send an email alert */
function emailalert($message)
{
global $em_enable, $email_account;
if(!$em_enable)
{
return;
}
error_log($message, 1, $email_account);
}
/* Validate user */
function validateUser($user, $pass, $valid_users)
{
$valid = false;
foreach($valid_users as $id => $user_obj)
{
if($id === $user && password_verify($pass , $user_obj->password_hash))
{
$valid=true;
break;
}
}
return $valid;
}
/* Get the file location for a given user */
function getFile($user)
{
global $valid_users;
$user_obj = $valid_users[$user];
return $user_obj->file;
}
/* Output resume content */
function displayResume($user, $ip)
{
/* Display the resume file */
$filename = getFile($user);
$input_file = fopen($filename, "r");
if(!$input_file)
{
error_log("Unable to open resume file! " . $filename,0);
exit(1);
}
echo fread($input_file,filesize($filename));
fclose($input_file);
$log_message = "Resume shown : ". $ip . " : " . $user . " : " . $filename;
error_log($log_message, 0);
emailalert($log_message);
updateDBView($ip, $user, $filename);
}
/* Check session validity */
function checkSession($username, $remoteip)
{
global $max_session_time, $session_timeout;
if(!isset($_SESSION['LoginTime']) || !isset($_SESSION['LoginStatus']))
{
return false;
}
$currenttime = time();
if($currenttime - $_SESSION['LoginTime'] > $max_session_time)
{
error_log("Maximum session time exceeded! : " . $remoteip . " : " . $username,0);
return false;
}
if($currenttime - $_SESSION['LoginStatus'] < $session_timeout)
{ //Session still valid
return true;
}
error_log("Session time out : " . $remoteip . " : " . $username ,0);
/* Session timeout */
return false;
}
/* Destroy the existing session */
function destroySession()
{
error_log("destroySession(): Clearing active session ", 0);
$sess_name = session_name();
$sessioncookie = "Set-Cookie: " . $sess_name . "=deleted;" . " expires=Thu, 01 Jan 1970 00:00:00 GMT; path=/; secure; HttpOnly; SameSite=Strict" ;
//Clear all session variables
$_SESSION = array();
//Destroy the session
session_destroy();
//clear any session cookie
header_remove("Set-Cookie");
header($sessioncookie);
}
/* Set security headers */
function secureHeaders()
{
header('Content-Type: text/html; charset=UTF-8');
header('Cache-control: no-store');
header('X-Content-Type-Options: nosniff');
header('X-Frame-Options: DENY');
header('X-XSS-Protection: 1; mode=block');
header('Content-Security-Policy: default-src \'self\'; frame-ancestors \'self\'; base-uri \'self\'; form-action \'self\'; report-uri /csprp/; ');
header('Referrer-Policy: same-origin');
}
/* Check the submitted captcha */
function checkCaptcha()
{
global $captcha_timeout, $captcha_enable;
if(!$captcha_enable)
{
return true;
}
if( !isset($_SESSION['CAPCREATE']) || !isset($_SESSION['captcha']) || !isset($_POST['ccode']) )
{//captcha not set
return false;
}
$currenttime = time();
if($currenttime - $_SESSION['CAPCREATE'] > $captcha_timeout)
{
return false;
}
$captcha_value = '**********';
$captcha_value = $_SESSION['captcha'];
$submitted_captcha = $_POST['ccode'];
if(strcasecmp($captcha_value, $submitted_captcha) === 0)
{
return true;
}
return false;
}
$remoteip="";
if(isset($_SERVER['HTTP_X_REAL_IP']))
{
$remoteip = " remote ip/real ip : " . $_SERVER['REMOTE_ADDR'] . " : " . $_SERVER['HTTP_X_REAL_IP'];
}
else
{
$remoteip = $_SERVER['REMOTE_ADDR'];
}
/* Check that request method is set */
if(!isset($_SERVER['REQUEST_METHOD']))
{
error_log("Request Method not set : " . $remoteip ,0);
exit(1);
}
/* Allow only GET and POST */
if(strcasecmp("post", $_SERVER['REQUEST_METHOD']) != 0 && strcasecmp("get", $_SERVER['REQUEST_METHOD']) != 0)
{
error_log("Invalid HTTP method : " . $_SERVER['REQUEST_METHOD'] . " : " . $remoteip ,0);
exit(1);
}
secureHeaders();
session_start();
$form_submitted = false;
$error_message = "";
$username = "";
/* Handle HTTP POST */
if(strcasecmp("post", $_SERVER['REQUEST_METHOD']) == 0 )
{
if( !isset( $_SESSION['LoginStatus'] ) )
{ /* Handle login */
$form_submitted = true;
if(!isset($_POST['userid']) || !isset($_POST['password']))
{//userid and password not set
error_log("HTTP POST submission : " . $remoteip . " : userid or password is not set",0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
$username = $_POST['userid'];
$password = $_POST['password'];
if(checkCaptcha())
{
if(validateUser($username, $password,$valid_users))
{
session_regenerate_id();
$_SESSION['LoginTime'] = time();
$_SESSION['LoginStatus'] = time();
$_SESSION['LoginUser'] = $username;
displayResume($username, $remoteip);
exit(0);
}
else
{
/* Generate a sleep value between 200 million ns to 999 million ns */
$sleep_val = rand(200000000,999999999);
/* Sleep for random ns */
time_nanosleep(0, $sleep_val);
$error_message = "Invalid Userid and Password !";
$log_message = "Failed login attempt : " . $remoteip . " : " . $username . " : " . $password;
error_log($log_message, 0);
updateDBFailLogin($remoteip, $username, $password);
emailalert($log_message);
}
}
else
{
$error_message = "Invalid Captcha !";
error_log("Invalid Captcha : " . $remoteip . " : " . $username , 0);
}
}
else
{ /* Already login and login session exists */
if(isset($_SESSION['LoginUser']))
{
$username = $_SESSION['LoginUser'];
}
if(!checkSession($username, $remoteip))
{//Invalid session
destroySession();
error_log("Login Status session expire redirecting : " . $remoteip . " : " . $username , 0);
header("Location: " . $rurl);
exit(0);
}
if(!isset($_SERVER['REQUEST_URI']))
{
error_log("Request uri not set !", 0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
$url = $_SERVER['REQUEST_URI'];
$query = parse_url($url,PHP_URL_QUERY);
if(!$query)
{//Invalid query
error_log("Invalid url query string ! : " . $remoteip . " : " . $username , 0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
$query = urldecode($query);
if($query === "q=refresh")
{
error_log("q=refresh received : " . $remoteip . " : " . $username, 0);
http_response_code(200); //Send HTTP 200 ok
echo "ok";
exit(0);
}
else if($query === "q=update")
{
//update session activity time
error_log("q=update received : ". $remoteip . " : " . $username, 0);
$_SESSION['LoginStatus'] = time();
http_response_code(202); //Send HTTP 202 accepted
echo "ok";
exit(0);
}
else
{
error_log("Invalid url query string ! : " . $remoteip . " : " . $username, 0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
}
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" type="text/css" href="../css2/main.css">
<link rel="stylesheet" type="text/css" href="rsm.css">
<script type="text/javascript" src="rs.js" async></script>
<title>View Resume - 夜空思间登录</title>
</head>
<body>
<header class="mhead">
<h1 class="mhead">Night Hour</h1>
<span class="mhead">Reading under a cool night sky ... 宁静沉思的夜晚 ...</span>
<nav class="tnav">
<ul class="tul" id="tul">
<li class="icon" id="navicon"><a href="#">☰ Menu</a></li>
<li><a href="https://www.nighthour.sg/">Home</a></li>
<li><a href="https://www.nighthour.sg/articles/">Articles</a></li>
<li><a href="/projects.html">Projects</a></li>
<li><a href="/humour.html">Humour</a></li>
<li><a href="/games/">Games</a></li>
<li><a href="/links.html">Links</a></li>
<li><a href="/apps/login.php">Login</a></li>
<li><a href="/zh/index.html">中文</a></li>
<li><a href="/resume/">Resume</a></li>
<li><a href="/about.html">About</a></li>
</ul>
</nav>
</header>
<div class="content">
<section>
<article class="art">
<h2>Login to View Resume - 履历表登录</h2>
<div class="rs_center">
<p>
<form class="rms_form" action="<?php echo $relative_url; ?>" accept-charset="utf-8" method="post" enctype="application/x-www-form-urlencoded" id="rs_form">
<div class="rms_form_row">
<label>Username</label><br><input type="text" name="userid" id="rs_userid" placeholder="Username" maxlength="50" size="30" required>
</div>
<div class="rms_form_row">
<label>Password</label><br><input type="password" name="password" id="rs_password" autocomplete="off" placeholder="Password" maxlength="100" size="30" required>
</div>
<br>
<?php if($captcha_enable): ?>
<div class="rms_form_row">
<?php
$ranvalue = mt_rand(1000, 99999);
$catch_img_src = "/captcha.php" . "?" . $ranvalue;
?>
<div class="rms_img_d">
<img id="captchaimg" class="rs_cap_img" src="<?php echo $catch_img_src;?>">
<button id="creload" type="button">Reload Captcha</button> <br>
</div>
</div>
<br>
<div class="rms_form_row">
<strong>I am not a robot, enter the text above :</strong><br>
<input name="ccode" id="captcha_code" size="30" type="text"> <br>
</div>
<?php endif; ?>
<div class="rms_form_row">
<input type="submit" value="Log in" id="login">
</div>
</form>
</p>
<p class="error" id="msg">
<?php
if($form_submitted)
{
echo $error_message . " <br>";
}
?>
</p>
</div>
<br>
<p>
If you are a recruiter and is interested to view my online resume, feel free to drop me an email at
<a href="/contact.html" target="_blank">Contact Me</a>. A modern browser like firefox, chrome or edge is needed to view the page properly.
</p>
<p>
如果您是一名网上招聘人员,想看看我的履历。您可以通过 <a href="/contact.html" target="_blank">Contact Me</a>,来请求登录帐号。请用新版本的网络浏览器像,firefox, chrome, 或者 edge 来查看这网页。
</p>
</article>
</section>
</div>
<footer class="pgbtm">
<p class="dclm">
Disclaimer: The content of this website is my personal opinion and is provided as is, without any warranties or fitness of any kind.
Use it at your own risk. The author will not be responsible for any omissions, mistakes, errors, any form of direct or indirect losses
arising from the use of this website, including any third party websites, third party content or applications, referred, embedded or used here.
</p>
<p class="dclm">
Privacy: The author respects user privacy. Information collected by this website will only be used to provide services to the users of this site. This website may utilize cookies, third party services such as advertisements, site analytics etc... Such third party services may track and collect user information which are subjected to the terms and policies of the third party providers. This website contains links to other external websites. These external websites have their own terms and policies. By continuing to browse this site, you agree to and accept the policies and terms specified by this site.
</p>
© 2020 Ng Chiang Lin, 强林<p>To send feedback or to contact the author, <a href="https://www.nighthour.sg/contact.html">Contact/Feedback</a> </p>
<p>
<a href="https://creativecommons.org/licenses/by-sa/4.0/" rel="license noopener noreferrer" target="_blank"><img alt="Creative Commons License" src="/images1/88x31.png"></a><br>This work is licensed under a <a href="https://creativecommons.org/licenses/by-sa/4.0/" rel="license noopener noreferrer" target="_blank">Creative Commons Attribution-ShareAlike 4.0 International License</a>.
</p>
</footer>
</body>
</html>
|
For better organization, we create a seperate directory to hold the resume viewer php script, the CSS and javascript files. This can be placed in our web server public document root. In this case, I am using a directory called "resume". Do secure this directory and the files inside with proper ownership and permission.
Take note that the actual resume files (containing our personal data) should be stored securely in a private location on our server. These files should not be placed inside the publicly accessible web document root.
The bulk of the php code are in the top portion of the script. In the lower portion, it is mainly the html 5 markups for the login form. The html 5 is customized for my nighthour.sg website. If you are using this for your own website, you need to modify accordingly.
We define a User class with 3 properties, a userid, a password hash and a file (the resume file) that they can view. For password hashing, we use the bcrypt hash generated by our utility described earlier. A $valid_users array is defined and we initalize the authorized users in this array. The code snippet is shown below.
class User
{
public $userid;
public $password_hash;
public $file;
function __construct($userid, $password_hash, $file)
{
$this->userid = $userid;
$this->password_hash = $password_hash;
$this->file = $file;
}
}
$valid_users = array (
"hr01" => new User("hr01",
'$2y$10$OoeJGygHV0eP8VLuiiGzFuI/UzpkV3PAKPB2RexhUcWOzjx7g2lGG',
'/opt/resume/resume.html'),
"hr02" => new User("hr02",
'$2y$10$tXRE07sSvAdOlxZyyZLgceozQuzC0QCiPcXqyZIrN9ec.G3NtvKxi',
'/opt/resume/resume-10-20.html'),
"hr03" => new User("hr03",
'$2y$10$lPnIVt5kBRoJHgxLtb0h5u8vK8Nw3FKEY5ox38xlbNinkZx1PSZR2',
'/opt/resume/resume-10-12.html'),
"myuser01" => new User("myuser01",
'$2y$10$KVOfnXtbU3A4GA7cqKgL/e5s9Nxn/MtFxBCA6lJUrEky8ptVKAULq',
'/opt/resume/resume-10-15.html')
);
Notice that our resume files are stored in the "/opt/resume/" directory. Make sure that you secure this directory and the individual resume files with the proper ownership and permission. In my server setup, I am using nginx web server with php-fpm. Nginx is running under the nginx user while php-fpm is running under the www-data user.
To secure the ownership and permissions on my resume directory and files, I can use the following commands. This sets it so that only the php script have permission to access these files.
chmod 750 /opt/resume
chown www-data: /opt/resume/*
chmod 640 /opt/resume/*
A number of toggling flags and variables are defined in the script.
$db_enable = true; //set whether mariadb database is enabled
$em_enable = true; // set whether email alert is enabled
$captcha_enable = true; // set whether captcha is enabled
$email_account = "my-resume-alert001@nighthour.sg";
$rurl = "https://nighthour.sg/resume/";
$captcha_timeout = 900;
$max_session_time = 1800; // maximum view time 30 minutes
$session_timeout = 300; //time out after 5 mins of no activity
The 3 boolean values, $db_enable, $em_enable and $captcha_enable defines whether our statistics are logged into a mariadb database (requires mariadb to be running), whether email alerts are enabled (requires smtp server), and whether captcha is available. The $max_session_time defines a maximum time that a user can view the resume file. $session_timeout defines the maximum amount of time of no activity before a user is logged out.
If a mariadb database server is available. You can create the database schema using the following commands. Note, replace the database user password with your own complex and long password.
create database resumedb1;
use resumedb1
create table resumeview
(
id INT NOT NULL PRIMARY KEY AUTO_INCREMENT,
time TIMESTAMP,
ip VARCHAR(64),
username varchar(256),
file varchar(512)
);
create table failedlogin
(
id INT NOT NULL PRIMARY KEY AUTO_INCREMENT,
time TIMESTAMP,
ip VARCHAR(64),
username varchar(256),
password varchar(256)
);
CREATE USER 'resumeuser01'@'localhost' IDENTIFIED BY 'XjDxIzdk#V8>Q%+9a!1BVqceFusFP-qN@dQgNE3uVy4JbULm' REQUIRE SSL;
GRANT INSERT on resumedb1.resumeview to 'resumeuser01'@'localhost';
GRANT INSERT on resumedb1.failedlogin to 'resumeuser01'@'localhost';
FLUSH PRIVILEGES;
The database user is only granted the INSERT privilege for the two tables. The resumeview table will log statistics for when a resume file is viewed. The failedlogin table will record fail login attempts. Notice that SSL is required for connecting to the database. The mariadb database server is set up with TLS/SSL connection.
On the resume viewer script, the following function shows how a PDO connection to the database is obtained. Notice that we are using client TLS certificates. The option MYSQL_ATTR_SSL_VERIFY_SERVER_CERT is disabled, this is due to the CN of the server certificate not matching the hostname. I didn't use a matching CN name when creating the server certificate and is lazy to regenerate it again. In this case, the risk is not high, since the client and server are located on the same machine.
All the database insertion operations are done securely through the use of prepared statements. This will prevent SQL injection attacks.
function getDatabasePDO()
{
$host = '127.0.0.1';
$db = 'resumedb1';
$user = 'resumeuser01';
$pass = 'XjDxIzdk#V8>Q%+9a!1BVqceFusFP-qN@dQgNE3uVy4JbULm';
$charset = 'utf8';
$dsn = "mysql:host=$host;dbname=$db;charset=$charset";
$opt = [
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION,
PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC,
PDO::ATTR_EMULATE_PREPARES => false,
PDO::MYSQL_ATTR_SSL_KEY =>'/opt/phpcerts/client-key.pem',
PDO::MYSQL_ATTR_SSL_CERT=>'/opt/phpcerts/client-cert.pem',
PDO::MYSQL_ATTR_SSL_CA =>'/opt/phpcerts/ca-cert.pem',
PDO::MYSQL_ATTR_SSL_VERIFY_SERVER_CERT => false
];
$pdo = new PDO($dsn, $user, $pass, $opt);
return $pdo;
}
When a user submit his or her credentials, the following snippet handles the HTTP POST request.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 |
/* Handle HTTP POST */
if(strcasecmp("post", $_SERVER['REQUEST_METHOD']) == 0 )
{
if( !isset( $_SESSION['LoginStatus'] ) )
{ /* Handle login */
$form_submitted = true;
if(!isset($_POST['userid']) || !isset($_POST['password']))
{//userid and password not set
error_log("HTTP POST submission : " . $remoteip . " : userid or password is not set",0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
$username = $_POST['userid'];
$password = $_POST['password'];
if(checkCaptcha())
{
if(validateUser($username, $password,$valid_users))
{
session_regenerate_id();
$_SESSION['LoginTime'] = time();
$_SESSION['LoginStatus'] = time();
$_SESSION['LoginUser'] = $username;
displayResume($username, $remoteip);
exit(0);
}
else
{
/* Generate a sleep value between 200 million ns to 999 million ns */
$sleep_val = rand(200000000,999999999);
/* Sleep for random ns */
time_nanosleep(0, $sleep_val);
$error_message = "Invalid Userid and Password !";
$log_message = "Failed login attempt : " . $remoteip . " : " . $username . " : " . $password;
error_log($log_message, 0);
updateDBFailLogin($remoteip, $username, $password);
emailalert($log_message);
}
}
else
{
$error_message = "Invalid Captcha !";
error_log("Invalid Captcha : " . $remoteip . " : " . $username , 0);
}
}
else
{ /* Already login and login session exists */
if(isset($_SESSION['LoginUser']))
{
$username = $_SESSION['LoginUser'];
}
if(!checkSession($username, $remoteip))
{//Invalid session
destroySession();
error_log("Login Status session expire redirecting : " . $remoteip . " : " . $username , 0);
header("Location: " . $rurl);
exit(0);
}
if(!isset($_SERVER['REQUEST_URI']))
{
error_log("Request uri not set !", 0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
$url = $_SERVER['REQUEST_URI'];
$query = parse_url($url,PHP_URL_QUERY);
if(!$query)
{//Invalid query
error_log("Invalid url query string ! : " . $remoteip . " : " . $username , 0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
$query = urldecode($query);
if($query === "q=refresh")
{
error_log("q=refresh received : " . $remoteip . " : " . $username, 0);
http_response_code(200); //Send HTTP 200 ok
echo "ok";
exit(0);
}
else if($query === "q=update")
{
//update session activity time
error_log("q=update received : ". $remoteip . " : " . $username, 0);
$_SESSION['LoginStatus'] = time();
http_response_code(202); //Send HTTP 202 accepted
echo "ok";
exit(0);
}
else
{
error_log("Invalid url query string ! : " . $remoteip . " : " . $username, 0);
destroySession();
header("Location: " . $rurl);
exit(1);
}
}
}
|
It checks the captcha if it has been enabled. If the userid and password is validated successfully, 3 session variables are set. The 3 session variables are LoginTime, LoginStatus and LoginUser. LoginTime is the time that the user has logged in. It is used for tracking the maximum time that a user can view the resume file. The LoginStatus is last activity time of the user, it is updated when user activity is detected. LoginUser stores the userid.
Notice that the session id is regenerated using the function session_regenerate_id() when a login is successful. This is to mitigate against Session Fixation attack. By changing the session id, it prevents an attacker from using a known session id prior to user login.
Besides handling a user login, HTTP POST is also used for refresh and update request. A refresh request is sent from the client browser to check for session validity, ensuring that a user has not exceeded the maximum no activity time. An update is sent when activity is detected on the client browser. It updates the last activity time of the user.
Throughout the script, there are ample logging that can be used for monitoring purposes. We logged errors such as failed logins, failed captchas etc... The ip address of the client is captured in the logs. This will allow an administrator to react and take measures againts an abusive or malicious remote ip addresses.
Take note that when a failed login occurs, both the username and password will be logged. There can be some security concerns about logging password even if it is the wrong password. For the case here, since I own the data and I am the one accessing the logs and doing the monitoring; there is no concern about sensitive information being leaked out to third party administrators. In other circumstances, you may to review whether certain sensitive content should be logged.
The displayResume() function is called to show the resume file for a particular logged in user. It reads in the resume file from the filesystem and send its content as output. The following shows the code snippet.
/* Output resume content */
function displayResume($user, $ip)
{
/* Display the resume file */
$filename = getFile($user);
$input_file = fopen($filename, "r");
if(!$input_file)
{
error_log("Unable to open resume file! " . $filename,0);
exit(1);
}
echo fread($input_file,filesize($filename));
fclose($input_file);
$log_message = "Resume shown : ". $ip . " : " . $user . " : " . $filename;
error_log($log_message, 0);
emailalert($log_message);
updateDBView($ip, $user, $filename);
}
We will go through the code for the javascript protection, prs.js. This javascript file needs to be included in the html resume file. The script will check for timeout as well as send user activity updates when browser activity is detected on the resume page.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 | (function()
{
const debug = false;
const REFRESH_TIME = 1000 * 60;
const URL_PATH = "/resume/";
const MAIN_URL = "https://" + window.location.hostname + URL_PATH;
const REFRESH_URL = "https://" + window.location.hostname + URL_PATH + "?q=refresh";
const UPDATE_URL = "https://" + window.location.hostname + URL_PATH + "?q=update";
const THROTTLE_TIME = 1000 * 60;
const MAX_VIEW_TIME = 1000 * 60 * 30;
const DOC_TITLE = "View Resume - 夜空思间登录";
let throttle = false;
let refresh_resp = false;
onload=init;
function init()
{
setTimeout(
()=> {
refresh(REFRESH_URL);
},
REFRESH_TIME
);
setTimeout(
()=>{
window.location.replace(MAIN_URL);
verifyDocumentClear();
},
MAX_VIEW_TIME
);
document.onmousemove = detectMouseActivity;
window.addEventListener('scroll', detectScrollActivity);
}
function detectMouseActivity(event)
{
if(debug)
{
console.log("Mouse movement event detected ! " + event.clientX + " : " + event.clientY);
}
updateActivity();
}
function detectScrollActivity(event)
{
if(debug)
{
console.log("Scrolling detected ! ");
}
updateActivity();
}
/* Clears the document if the title is unexpected */
function clearDocument()
{
if(document.title !== DOC_TITLE)
{
document.open();
document.write("Network disconnection application timeout.");
document.close();
}
}
/* Verfies that the sensitive document is no longer displayed */
function verifyDocumentClear()
{
setTimeout(clearDocument, 5000);
}
function updateActivity()
{
if(throttle) return;
throttle = true;
setTimeout(
()=>{
throttle = false;
},
THROTTLE_TIME
);
refresh(UPDATE_URL);
}
function handleRefreshResponse(xhr)
{
if(xhr.readyState === XMLHttpRequest.DONE)
{
refresh_resp = true;
if (xhr.status === 200 || xhr.status === 202)
{
if(debug)
{
console.log("valid response code");
}
if(xhr.responseText !== 'ok')
{
if(debug)
{
console.log("Invalid response text redirecting");
}
window.location.replace(MAIN_URL);
verifyDocumentClear();
}
}
else
{ // Any other response code
window.location.replace(MAIN_URL);
verifyDocumentClear();
}
}
}
function refresh(url)
{
if(url === REFRESH_URL)
{
refresh_resp = false;
if(debug)
{
console.log("Refreshing page");
}
/* Verify that we got a response back after 5 seconds, otherwise clear doc */
setTimeout(
() => {
if(!refresh_resp)
{
clearDocument();
}
},
5000
);
setTimeout(
()=> {
refresh(REFRESH_URL);
},
REFRESH_TIME
);
}
else
{
if(debug)
{
console.log("Updating activity");
}
}
xhr = new XMLHttpRequest();
if (!xhr)
{
if(debug)
{
console.log("Cannot create ajax objext falling back to location method");
}
window.location.replace(MAIN_URL);
}
xhr.onreadystatechange = () => { handleRefreshResponse(xhr) };
xhr.open('POST', url, true);
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xhr.send();
}
})();
|
The protection javascript, prs.js can be included in the html resume file just like any regular javascript file.
<script type="text/javascript" src="prs.js" async></script>
When the protection javascript starts up, it will register to detect mouse movements and scroll events. These indicate that there is activity on the resume page that is displayed. When activity is detected, an update will be sent via Ajax to the server. This will update the last activity time for the user. A throttle flag is used to ensure that one update can be sent per minute. This is to prevent lots of Ajax POSTs being sent to the server.
The protection javascript will check in with the server (refresh) very minute. If the user last activity time exceeds the maximum no activity period, the user session will be cleared and a redirection sent to the protection javascript. The javascript will redirect to the login page using a HTTP GET issued by window.location.replace().
The protection javascript will also try to check for network connection issues. If a network connection problem occurs and the resume page cannot be redirected to the login page, the javascript will clear the page. Take note that this detection uses the document title as a check. So the resume file needs to have a different html title from the resume viewer login page.
If you are creating a pdf version of the resume file for recruiters to download, do not include the protection javascript when converting to pdf. This is to prevent the javascript from being embedded into the pdf. You may also want to flatten the pdf by converting it to postscript and then converting it back to pdf again. Tools like pdftops and pstopdf can be used.
Just like we want to protect our personal data, companies and recruitment agencies will want to protect their HR systems from malicious files sent by fake job applicants. Dynamic scripts embedded inside pdf files can be mistaken as malicious payloads by security systems.
The full source code for bcrypthash.php (utility script), the index.php (resume viewer) and prs.js (protection javascript) are available at the Github link at the end of the article.
Testing
Ok, let's test out the secure online resume viewer. Create a nice html 5 resume and store it in the private location on the server. Make sure the html resume includes the protection javascript file in its html code. The protection javascript file and style sheet can be placed inside the resume directory together with the resume viewer php script. Configure the resume viewer php script with a user and password for accessing the resume file.
Start up the browser and navigate to the secure online resume viewer page.
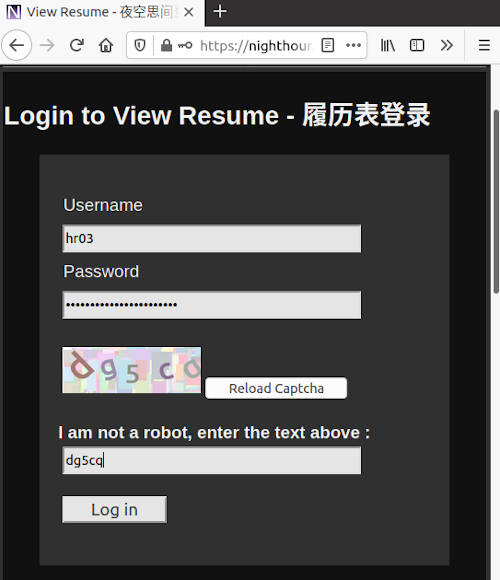
Upon successful login, the resume file will be displayed.
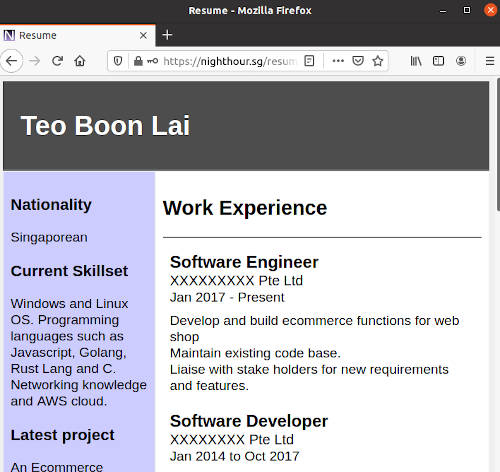
If there are no activity for about 5 minutes, you will be logged out automatically.
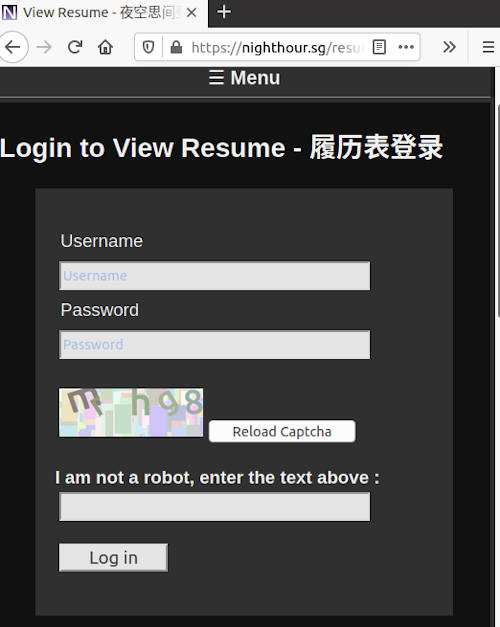
If the network got disconnected. The document will be cleared after about 1 minute.
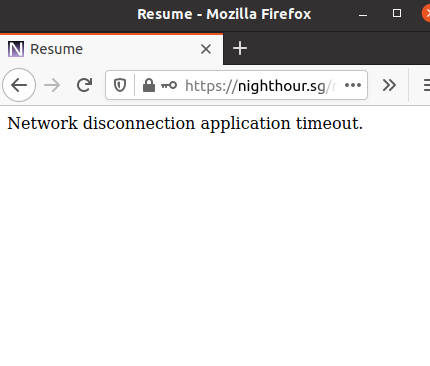
If you have enabled database logging. You should be able to query the database tables for statistics. For example, the failedlogin table can show failed login attempts. Take note that if captcha is enabled, the failedlogin will only capture failed logins that have cleared the captcha check successfully.
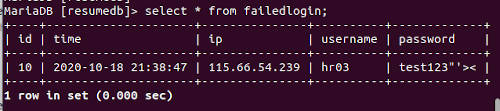
When email alerts are enabled. You will get emails notifying of resume being viewed and failed login attempts.
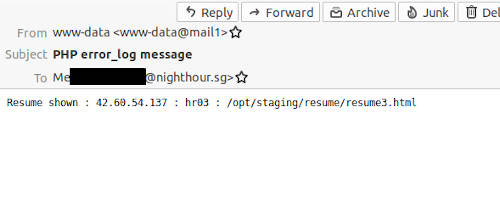
A Note on Security
The resume viewer script is flexible such that it can work without captcha, database or email alert. For security though, it is recommended all 3 be enabled. There are online tutorials on how to create php based captcha, so it is not really difficult to integrate captcha into your site. There are also online libraries for captcha if you do not want to write your own. I will recommend implementing/writing your own captcha, so that you can customize it. This is not only good for learning but also it reduces the risk of depending on third party code.
Take note that captcha is a mitigation against brute forcing, but it is not perfect. There are machine learning techniques that can crack captcha. One of the reason why I didn't include the captcha code for my website in this article is to keep the generation method secret. Yes, security through obscurity. If I publish my captcha code, it will make it easy for a malicious attacker to generate large amount of captcha dataset and then training a cracker with this dataset.
By not including the captcha code here, it makes it slightly harder for malicious hackers. Captcha can be coupled with other rate limiting techniques for better security defenses. It is also important not to confuse Captcha with proper authentication. Some non tech personnel may ask why have strong authentication when you already got a captcha.
Captcha is not a replacement for proper and strong authentication. Always use good and complex long passwords. For the resume viewer, there is no 2nd Factor Authentication implemented. This is a trade off I made. I may be giving access to external recruiters and HRs. It will be unreasonable of me to demand that they set up 2 factor authentication like a TOTP (Time based One time password) on their mobiles.
The email alerts and close monitoring will help mitigate against the lack of a 2 FA authentication. Some of the other protections like the time out when viewing the resume file depends on javascript. If a browser disable or do not support javascript, the resume viewer won't be able to automatically log out a user session.
A possible enhancement for the resume viewer is perhaps to check for javascript support before displaying the resume file.
When running php, make sure that the php session cookie settings are configured securely. In my debian 10 system, the version of php is 7.3. This version supports Same-Site session cookie which can help to mitigate against CSRF (Cross Site Request Forgery). I have also enabled the Httponly and Secure option for the session cookie. There are many other options in php.ini. Do run through it and harden all the settings accordingly.
Conclusion and Afterthought
This article shows how a simple, secure online resume viewer can be built quickly. It gives greater control over our own personal data and ensure that access is only granted to those that we trust.
In our digital age, data has become an important asset. Companies mine data to derive business insights, to discover consumers behaviour, to gain a business and commercial edge. Governments have come up with new regulations to protect the data and privacy of individuals and consumers. In Singapore, we have our own Personal Data Protection Act and an government agency created for this.
Individuals need to be aware of data privacy and security issues, and take the necessary steps to protect our own personal data and privacy.
Useful References
- OWASP Top 10, The top 10 vulnerabilities that applications should prevent and avoid.
- Personal Data Protection Commission Singapore, Singapore's main authority on the protection of personal data.
- Web Application Security Headers, an article on the various HTTP Security headers that can be set for an application.
- Creating a Finite State Php Rate Limiter, an article on setting up a php rate limiter that can throttle requests to a web resource.
- Building a UDP Token Bucket Rate Limit Server, an article on setting up a Token Bucket Rate Limiter.
- Rate Limiting with NGINX and NGINX Plus, a blog post about rate limiting http requests with Nginx.
- AweSome Captcha, a listing of captcha libraries including php based captcha and crackers for captcha.
- How to break a CAPTCHA system in 15 minutes with Machine Learning, an interesting article on cracking captcha with machine learning.
- Survive The Deep End: PHP Security, a useful resource on security for php web application. Some parts of the book is still incomplete but nevertheless, many of the completed sections are quite useful.
- Create your Professional/Educational resume using LaTeX, An interesting article about using Latex to create a resume.
The full source code for the scripts are available at the following Github link.
https://github.com/ngchianglin/VPS_MISC/tree/master/resume
If you have any feedback, comments, corrections or suggestions to improve this article. You can reach me via the contact/feedback link at the bottom of the page.